10 Essential React Native Component Patterns for Seamless Development
Hey there, fellow developers! If you’re diving into the world of React Native, you’re probably already familiar with its power to create stunning mobile applications using JavaScript. But to truly master React Native development, understanding and implementing efficient component patterns is key. In this post, I’ll walk you through 10 essential React Native component patterns that will streamline your development process and elevate your apps to the next level.
Whether you’re a seasoned React Native pro or just starting out on your journey, these patterns will equip you with the tools and techniques needed to build robust, scalable, and high-performance mobile applications. So grab your favorite coding beverage, buckle up, and let’s embark on this exciting journey together! Don’t worry, we’ll make sure to break down complex concepts into digestible bits along the way! Happy coding, and let’s dive into the wonderful world of React Native component patterns!
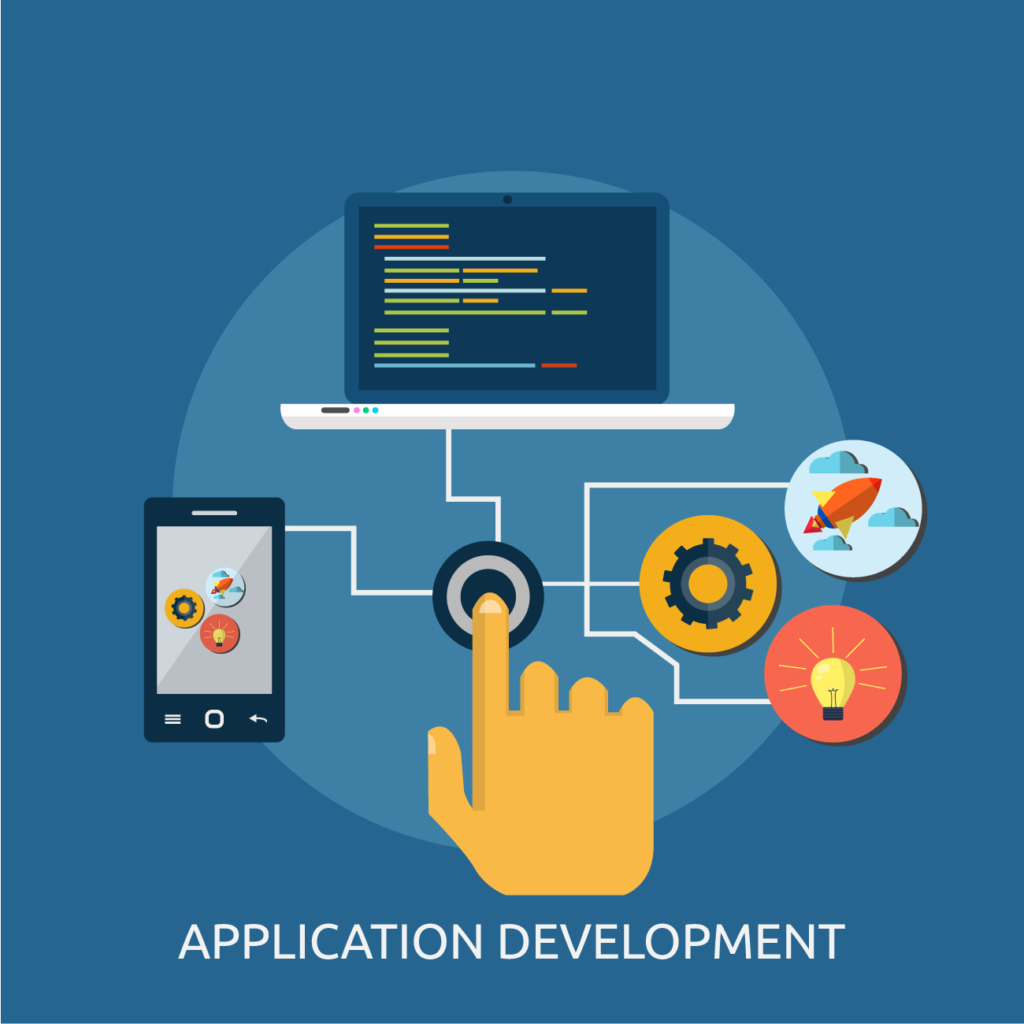
Introduction to React Native Component Patterns
Before we dive into the specifics, let’s briefly touch on what exactly React Native component patterns are. Think of them as tried-and-tested templates or blueprints for structuring and organizing your React Native UI components. By following these patterns, you ensure consistency, maintainability, and scalability in your codebase.
1. Container/Presenter Pattern
-
- What it is: This pattern separates the logic (container) from the presentation (presenter) of a component.
-
- Why it’s important: Enhances reusability and simplifies testing.
-
- Example: Utilize a container component to handle data fetching and state management, while the presenter component focuses solely on rendering UI elements.
2. Higher Order Components (HOCs)
-
- What they are: HOCs are functions that take a component and return a new enhanced component.
-
- Why they’re important: Enable code reuse and add additional functionality to components.
-
- Example: Create an HOC to add authentication or data fetching capabilities to multiple components across your app.
3. Render Props
-
- What they are: Render props are a technique for sharing code between React components using a prop whose value is a function.
-
- Why they’re important: Encourage component composition and provide flexibility in passing data and behavior down the component tree.
-
- Example: Pass a render prop to a component to dynamically render children based on the state or data.
4. Context API
-
- What it is: Context provides a way to pass data through the component tree without having to pass props down manually at every level.
-
- Why it’s important: Simplifies data sharing between components, especially in larger applications.
-
- Example: Use the Context API to provide theme information or user authentication status to all components in your app.
5. Controlled Components
-
- What they are: Controlled components are components that maintain their own state and update based on user input.
-
- Why they’re important: Ensure consistency between the UI and state, making it easier to manage form inputs and user interactions.
-
- Example: Create a controlled TextInput component that updates its value based on user input and triggers a callback function to update the parent component’s state.
6. Functional Components with Hooks
-
- What they are: Functional components are lightweight, stateless components that can use React Hooks to manage state and lifecycle.
-
- Why they’re important: Improve performance and readability of your code by leveraging hooks like useState and useEffect.
-
- Example: Refactor class-based components to functional components and utilize hooks for state management and side effects.
7. Presentational and Container Components
-
- What they are: Following the Separation of Concerns (SoC) principle, presentational components focus on rendering UI elements, while container components handle data and logic.
-
- Why they’re important: Promote code organization and maintainability by keeping concerns separate.
-
- Example: Divide your app’s components into presentational and container components, with presentational components being reusable across different parts of your app.
8. Lazy Loading
-
- What it is: Lazy loading is a technique for delaying the loading of components until they are needed.
-
- Why it’s important: Improves app performance by reducing initial load times and only loading components when necessary.
-
- Example: Use React.lazy() and Suspense to dynamically load components when they become visible to the user, such as in a tabbed navigation interface.
9. Error Boundaries
-
- What they are: Error boundaries are React components that catch JavaScript errors anywhere in their child component tree and display a fallback UI.
-
- Why they’re important: Prevents crashes and improves user experience by gracefully handling errors.
-
- Example: Wrap components that might throw errors, such as API requests or complex calculations, with an error boundary component to display a friendly error message to users.
10. Keyed Fragments
-
- What they are: Keyed fragments are a way to group multiple children without adding extra nodes to the DOM.
-
- Why they’re important: Improve performance by reducing unnecessary DOM nodes and optimizing component rendering.
-
- Example: Use keyed fragments when mapping over arrays of data to ensure efficient updates and minimize re-renders.
Conclusion
And there you have it—10 essential React Native component patterns to supercharge your development process! By incorporating these patterns into your projects, you’ll not only write cleaner and more maintainable code but also create apps that are more scalable and performant. So go ahead, dive in, and make the most out of your React Native component library!